Now that the game has a camera, a HUD and a Grass tile, we need to code some actual game logic. To start with, how about a class which handles all the game's data? Eventually this will need to be handled in a better way, but for now we can simply use PlayerPrefs to store the save game data, and create a singleton class to handle the game logic.
Keeping track of money
First I'll create a singleton class called SessionManager. This one will be responsible for storing the game's state, such as money, which blocks are where, etc.
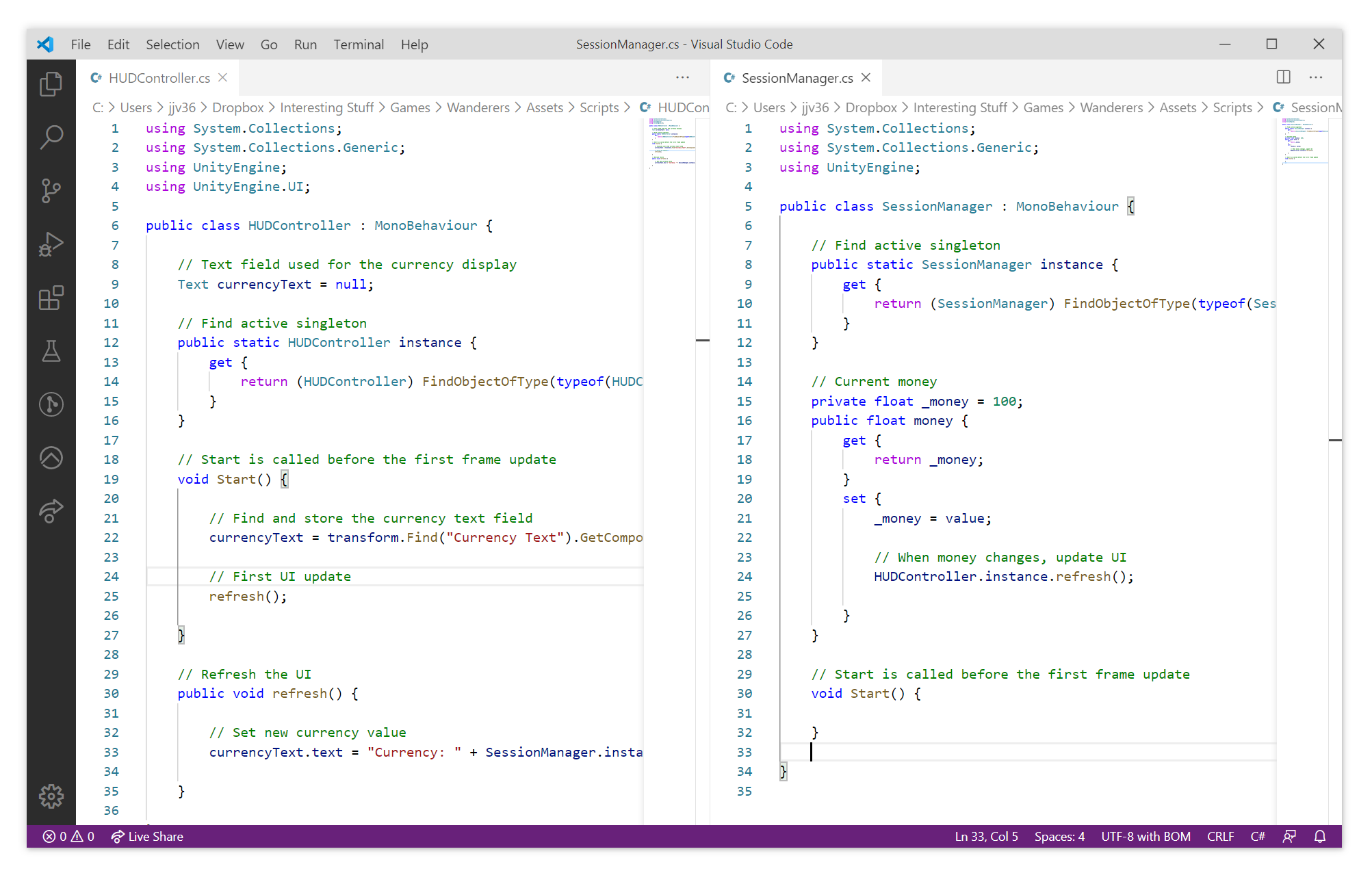
I added a instance
static variable which will reference the only instance of each of these classes. Using that, the SessionManager now has a money
property, and whenever that property changes it will trigger refresh()
on the HUDController
class. That will, in turn, fetch the money
from SessionManager
and display it on the HUD. Nice.
This means that, whenever the money
property changes, the HUD will automatically be updated to show the new value.
Spawning map tiles at runtime
I just realised we have a Grass tile, but we will also need a Placeholder tile. These tiles will be displayed around the surrounding area, allowing the user to click on them to expand the area. So, let's do that.
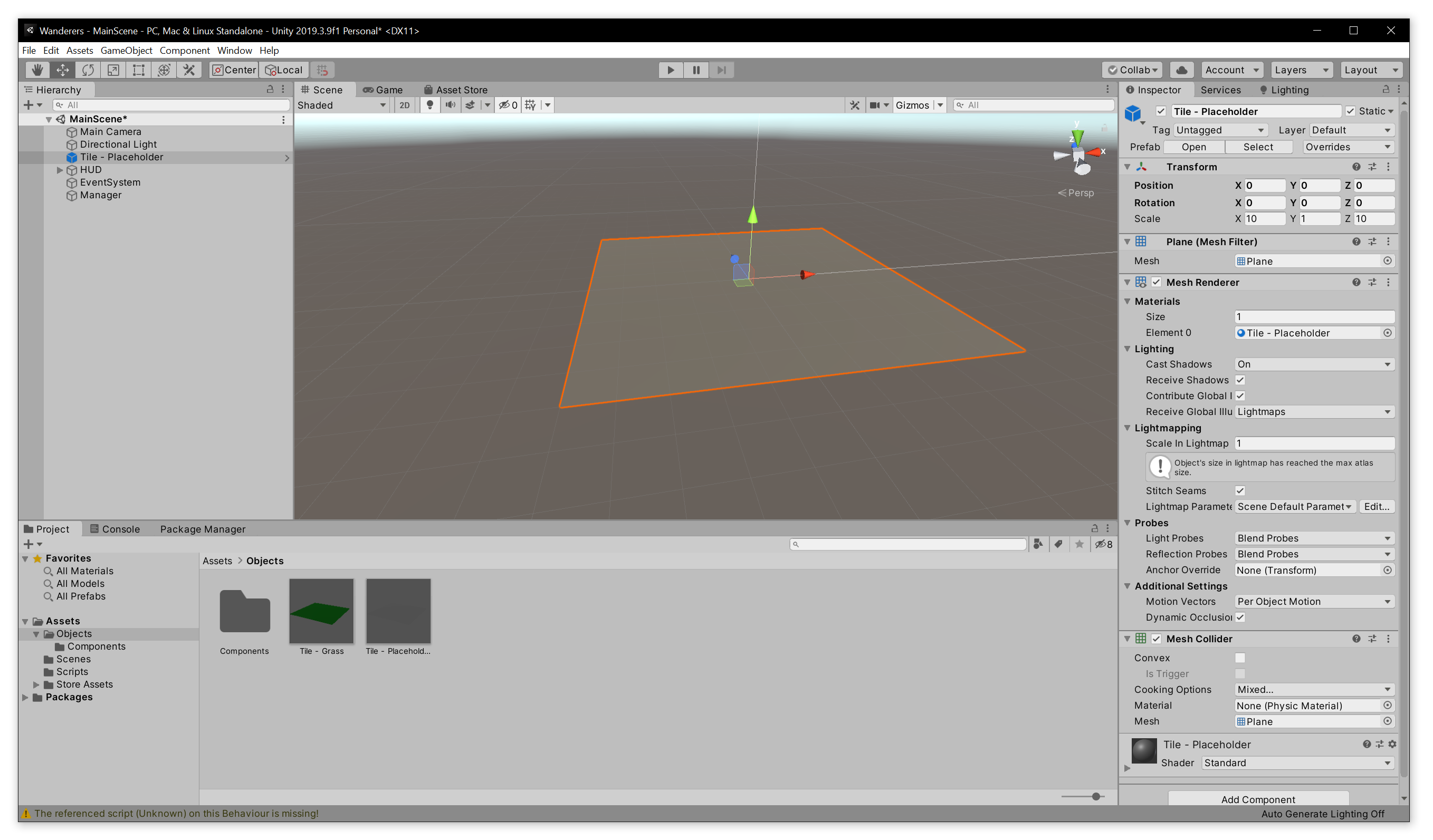
There we go. Now, we need to do the hard part of creating the map and loading in the prefabs at runtime. This will be done in the SessionManager class for now.
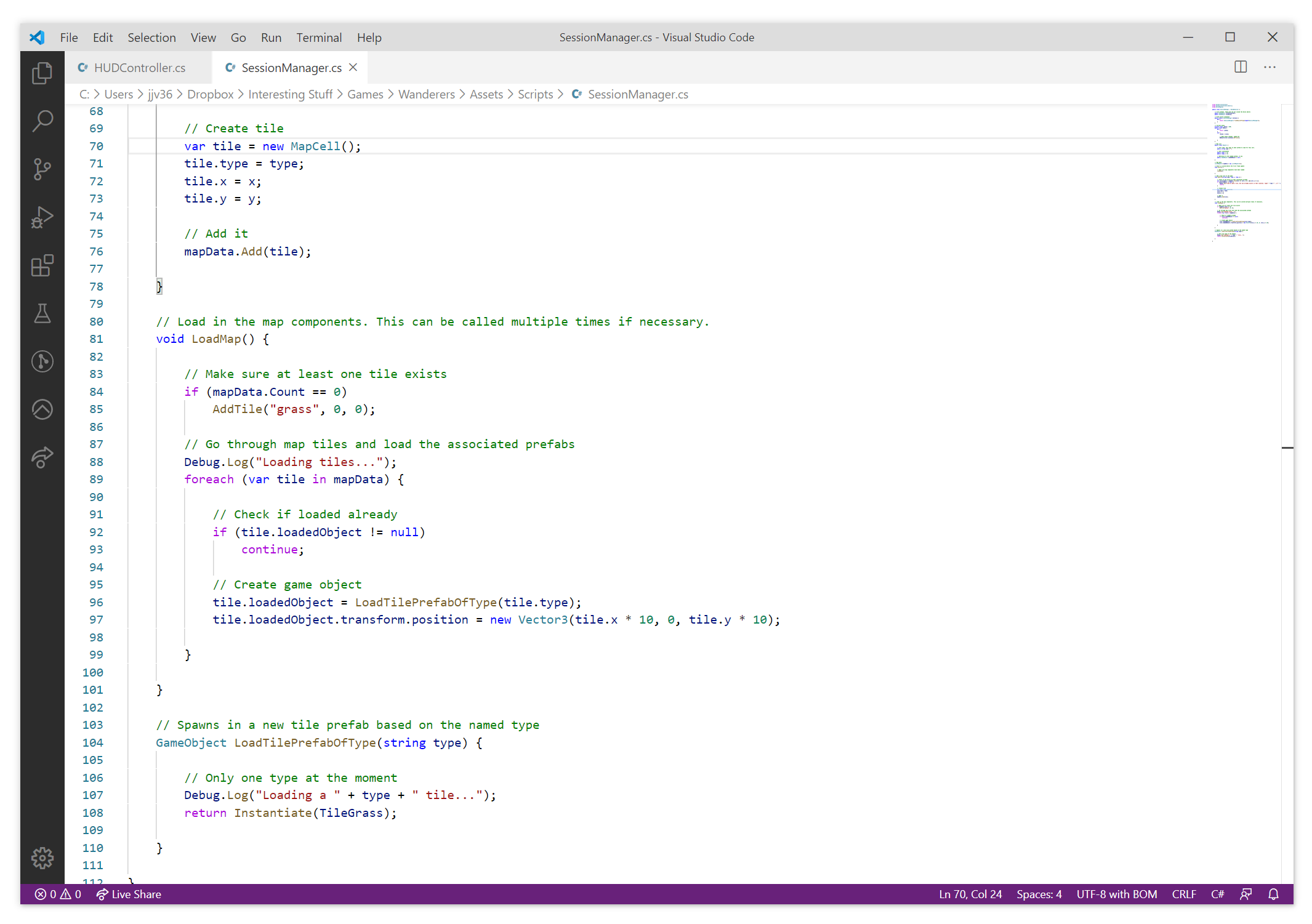
To achieve this, I added code to spawn map tile prefabs to the SessionManager
class. In Unity you can reference prefabs that don't exist in the scene by creating an uninitialized GameObject
variable, and then using the Inspector to assign prefab assets to those variables. Then, just call Instantiate(myPrefab)
to spawn in that prefab.
Showing the placeholder tiles
Placeholder tiles should be added around the map. The simplest way to do this, is to add some code to SessionManager
to ensure that the map is surrounded by placeholder tiles before loading in the map.
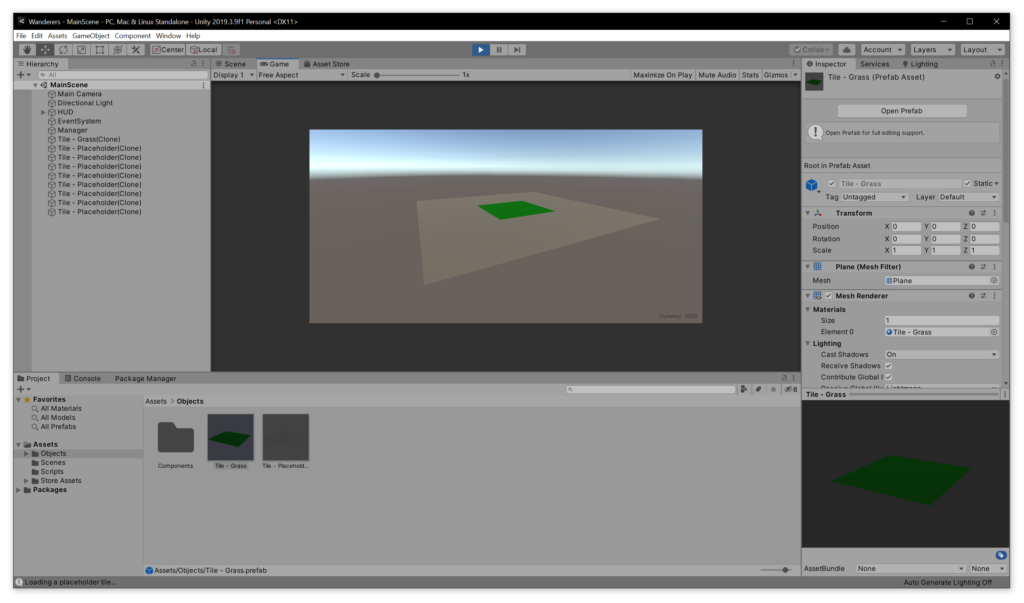
Nice! Now we have a blank scene, but when the game is run we have a grass tile surrounded by placeholder tiles. Next, let's allow the user to create tiles of their own. To make this simple, we'll have the user press the [E] key while looking directly at a placeholder tile, and that will turn it into a grass tile.
User-placeable grass tiles
We can use Input.GetKeyDown()
to listen for the key down event. For now, I'll add this code to the SessionManager
. Then, we'll need to do a raytrace from the center of the screen and see if it hits any placeholder tiles. If it does, we take some of the player's money and unload the placeholder tile's game object, and convert the tile into a grass tile.
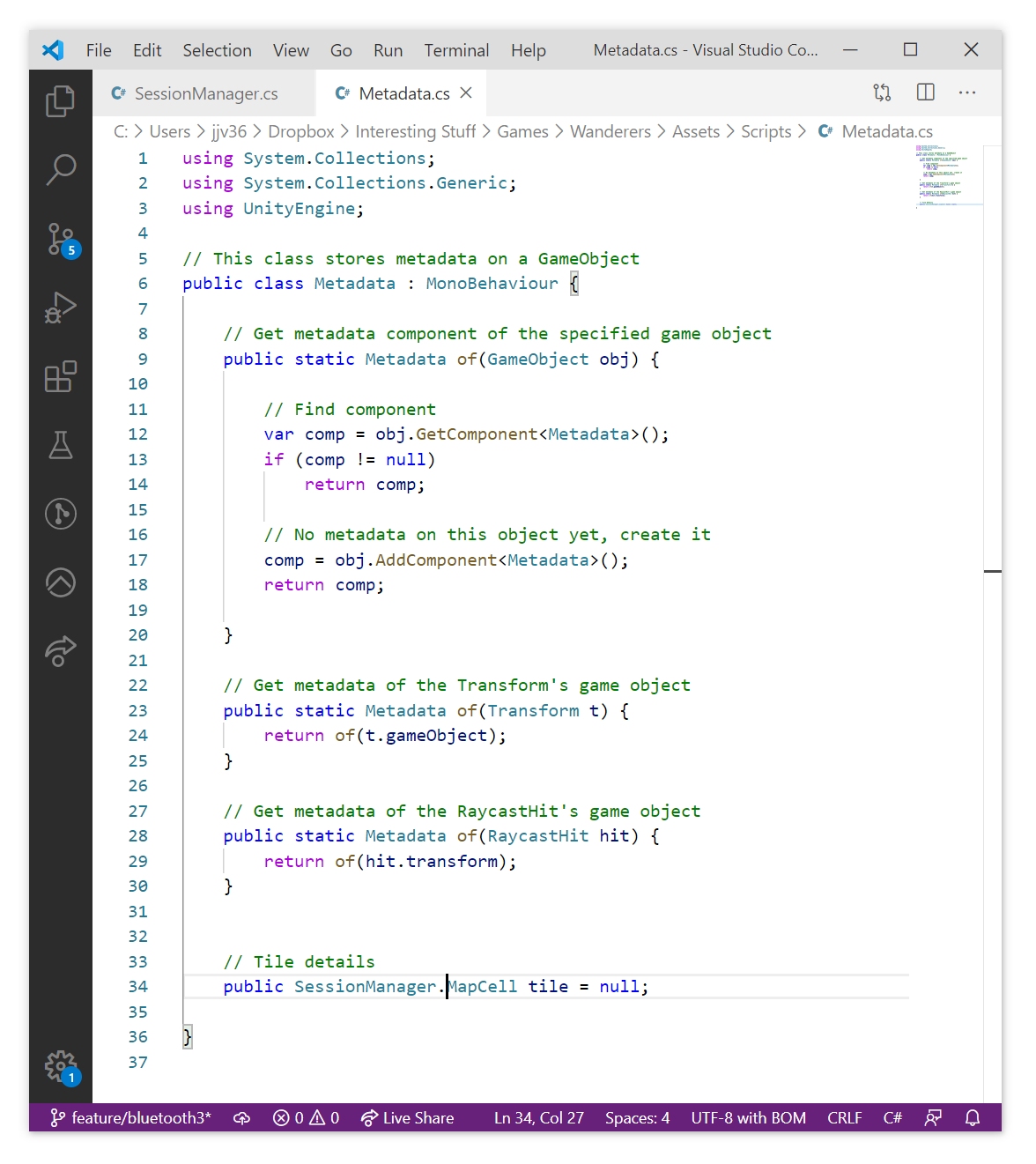
The class above is a simple Metadata
component, which can store details about a GameObject. In this case, we can check if a raytrace hit picked up a tile by checking Metadata.of(rayHit).tile != null
. That's nice and convenient.
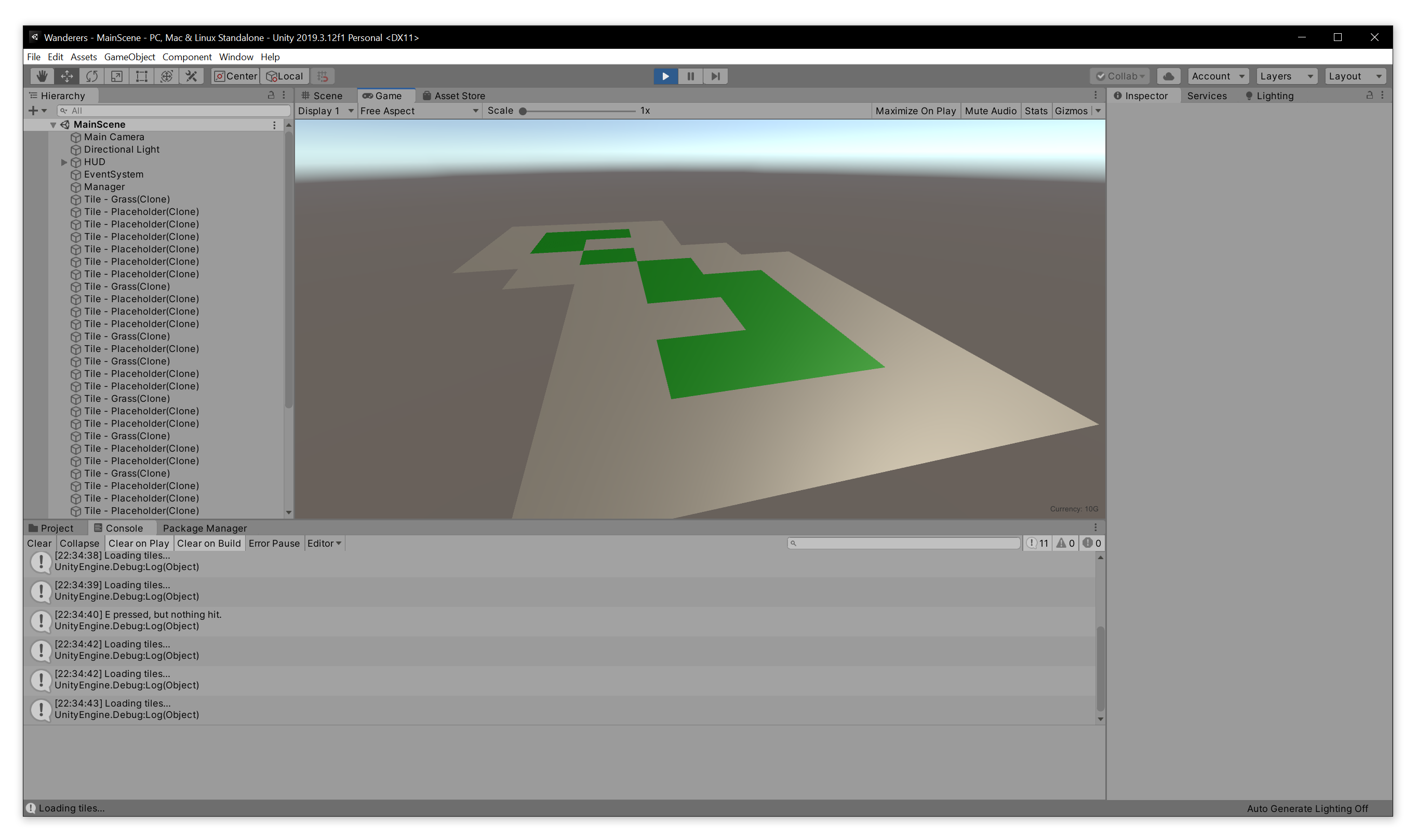
Nice! Now we have a map that can grow as the user spends money on it.